1)What is oops concept with example?
a-abstraction
b-incapsulation
c-polymorphism
d-inheritance.
Q-What is abstraction?
Ans:-Abstraction.Means hinding of data.
Abstraction is a process where you show only “relevant” data and “hide” unnecessary details of an object from the user. For example, when you login to your Amazon account online, you enter your user_id and password and press login, what happens when you press login, how the input data sent to amazon server, how it gets verified is all abstracted away from the you.
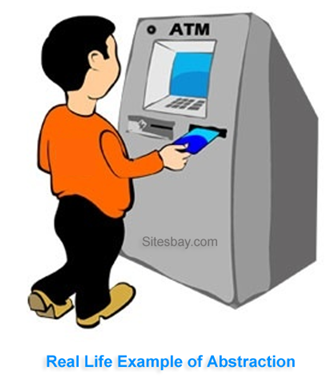
Ans:-Binding of data (variable and method ) in single unit.
Encapsulation can be achieved by: Declaring all the variables in the class as private and writing public methods in the class to set and get the values of variables
Q- What is overriding?
Ans-Declaring a method in subclass which is already present in super class is know as overriding.
Example-
Can we overload a static method in Java? (answer)
a-abstraction
b-incapsulation
c-polymorphism
d-inheritance.
Q-What is abstraction?
Ans:-Abstraction.Means hinding of data.
Abstraction is a process where you show only “relevant” data and “hide” unnecessary details of an object from the user. For example, when you login to your Amazon account online, you enter your user_id and password and press login, what happens when you press login, how the input data sent to amazon server, how it gets verified is all abstracted away from the you.
Advantages of Abstraction
- It reduces the complexity of viewing the things.
- Avoids code duplication and increases reusability.
- Helps to increase security of an application or program as only important details are provided to the user.
Another real life example of Abstraction is ATM Machine; All are performing operations on the ATM machine like cash withdrawal, money transfer, retrieve mini-statement…etc. but we can't know internal details about ATM.
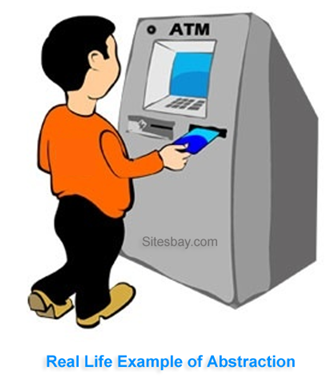
Note: Data abstraction can be used to provide security for the data from the unauthorized methods.
Note: In Java language data abstraction can achieve using class.
Example of Abstraction
class Customer { int account_no; float balance_Amt; String name; int age; String address; void balance_inquiry() { /* to perform balance inquiry only account number is required that means remaining properties are hidden for balance inquiry method */ } void fund_Transfer() { /* To transfer the fund account number and balance is required and remaining properties are hidden for fund transfer method */ }
How to achieve Abstraction ?
There are two ways to achieve abstraction in java
- Abstract class (0 to 100%)
- Interface (Achieve 100% abstraction)
Ans:-Binding of data (variable and method ) in single unit.
Encapsulation can be achieved by: Declaring all the variables in the class as private and writing public methods in the class to set and get the values of variables
Advantages of Encapsulation:
- Data Hiding: The user will have no idea about the inner implementation of the class. It will not be visible to the user that how the class is storing values in the variables. He only knows that we are passing the values to a setter method and variables are getting initialized with that value.
- Increased Flexibility: We can make the variables of the class as read-only or write-only depending on our requirement. If we wish to make the variables as read-only then we have to omit the setter methods like setName(), setAge() etc. from the above program or if we wish to make the variables as write-only then we have to omit the get methods like getName(), getAge() etc. from the above program
- Reusability: Encapsulation also improves the re-usability and easy to change with new requirements.
- Testing code is easy: Encapsulated code is easy to test for unit testing.
// Java program to demonstrate encapsulation public class Encapsulate { // private variables declared // these can only be accessed by // public methods of class private String geekName; private int geekRoll; private int geekAge; // get method for age to access // private variable geekAge public int getAge() { return geekAge; } // get method for name to access // private variable geekName public String getName() { return geekName; } // get method for roll to access // private variable geekRoll public int getRoll() { return geekRoll; } // set method for age to access // private variable geekage public void setAge( int newAge) { geekAge = newAge; } // set method for name to access // private variable geekName public void setName(String newName) { geekName = newName; } // set method for roll to access // private variable geekRoll public void setRoll( int newRoll) { geekRoll = newRoll; } } |
In the above program the class EncapsulateDemo is encapsulated as the variables are declared as private. The get methods like getAge() , getName() , getRoll() are set as public, these methods are used to access these variables. The setter methods like setName(), setAge(), setRoll() are also declared as public and are used to set the values of the variables.
The program to access variables of the class EncapsulateDemo is shown below:
public class TestEncapsulation { public static void main (String[] args) { Encapsulate obj = new Encapsulate(); // setting values of the variables obj.setName( "Harsh" ); obj.setAge( 19 ); obj.setRoll( 51 ); // Displaying values of the variables System.out.println( "Geek's name: " + obj.getName()); System.out.println( "Geek's age: " + obj.getAge()); System.out.println( "Geek's roll: " + obj.getRoll()); // Direct access of geekRoll is not possible // due to encapsulation // System.out.println("Geek's roll: " + obj.geekName); } } |
Output:
Geek's name: Harsh Geek's age: 19 Geek's roll: 51
Encapsulation vs Data Abstraction
- Encapsulation is data hiding(information hiding) while Abstraction is detail hiding(implementation hiding).
- While encapsulation groups together data and methods that act upon the data, data abstraction deals with exposing the interface to the user and hiding the details of implementation.
- Encapsulation is not providing full security because we can access private member of the class using reflection API, but in case of Abstraction we can't access static, abstract data member of a class.
import java.util.ArrayList;
import java.util.List;
abstract class Pet{
public abstract void makeSound();
}
class Cat extends Pet{
@Override
public void makeSound() {
System.out.println("Meow");
}
}
class Dog extends Pet{
@Override
public void makeSound() {
System.out.println("Woof");
}
}
import java.util.List;
abstract class Pet{
public abstract void makeSound();
}
class Cat extends Pet{
@Override
public void makeSound() {
System.out.println("Meow");
}
}
class Dog extends Pet{
@Override
public void makeSound() {
System.out.println("Woof");
}
}
Let's test How Polymorphism concept work in Java:
/**
*
* Java program to demonstrate What is Polymorphism
* @author Javin Paul
*/
public class PolymorphismDemo{
public static void main(String args[]) {
//Now Pet will show How Polymorphism work in Java
List<Pet> pets = new ArrayList<Pet>();
pets.add(new Cat());
pets.add(new Dog());
//pet variable which is type of Pet behave different based
//upon whether pet is Cat or Dog
for(Pet pet : pets){
pet.makeSound();
}
}
}
Output:
Meow
Woof
*
* Java program to demonstrate What is Polymorphism
* @author Javin Paul
*/
public class PolymorphismDemo{
public static void main(String args[]) {
//Now Pet will show How Polymorphism work in Java
List<Pet> pets = new ArrayList<Pet>();
pets.add(new Cat());
pets.add(new Dog());
//pet variable which is type of Pet behave different based
//upon whether pet is Cat or Dog
for(Pet pet : pets){
pet.makeSound();
}
}
}
Output:
Meow
Woof
Q- What is overriding?
Ans-Declaring a method in subclass which is already present in super class is know as overriding.
Example-
// A Simple Java program to demonstrate // method overriding in java // Base Class class Parent { void show() { System.out.println( "Parent's show()" ); } } // Inherited class class Child extends Parent { // This method overrides show() of Parent @Override void show() { System.out.println( "Child's show()" ); } } // Driver class class Main { public static void main(String[] args) { // If a Parent type reference refers // to a Parent object, then Parent's // show is called Parent obj1 = new Parent(); obj1.show(); // If a Parent type reference refers // to a Child object Child's show() // is called. This is called RUN TIME // POLYMORPHISM. Parent obj2 = new Child(); obj2.show(); } } |
Output:
Parent's show() Child's show()
Overriding vs Overloading :
- Overloading is about same method have different signatures. Overriding is about same method, same signature but different classes connected through inheritance.
- Overloading is an example of compiler-time polymorphism and overriding is an example of run time polymorphism.
Inheritance in Java
Inheritance is an important pillar of OOP(Object Oriented Programming). It is the mechanism in java by which one class is allow to inherit the features(fields and methods) of another class.
Important terminology:
- Super Class: The class whose features are inherited is known as super class(or a base class or a parent class).
- Sub Class: The class that inherits the other class is known as sub class(or a derived class, extended class, or child class). The subclass can add its own fields and methods in addition to the superclass fields and methods.
- Reusability: Inheritance supports the concept of “reusability”, i.e. when we want to create a new class and there is already a class that includes some of the code that we want, we can derive our new class from the existing class. By doing this, we are reusing the fields and methods of the existing class.
How to use inheritance in Java
The keyword used for inheritance is extends.
Syntax :
Syntax :
class derived-class extends base-class { //methods and fields }
Programmer salary is:40000.0 Bonus of programmer is:10000
Why use Inheritance ?
- For Method Overriding (used for Runtime Polymorphism).
- It's main uses are to enable polymorphism and to be able to reuse code for different classes by putting it in a common super class
- For code Re-usability
Advantage of inheritance
If we develop any application using concept of Inheritance than that application have following advantages,
- Application development time is less.
- Application take less memory.
- Application execution time is less.
- Application performance is enhance (improved).
- Redundancy (repetition) of the code is reduced or minimized so that we get consistence results and less storage cost.
All Data are copied from my website sitesbay.com
ReplyDeletehttps://www.sitesbay.com/java/java-abstraction