Quick Overview of Java String Class
- Java String Class is present in
java.util
package. - A string is a sequence of characters. But, it’s not a primitive data type but an object.
- String object is immutable in Java. So once created, we can’t change it.
- String has a shortcut way to create its objects using double quotes. These string objects are also called string literals.
- String is the only java class that supports operator overloading. We can use the ‘+’ operator to concatenate two strings.
- Java store string objects in a specific pre-defined area. String pool is the part of java heap space to store string literals.
- String is serializable in nature and implements
Serializable
interface. - Other interfaces implemented by String class are Comparable, CharSequence, Constable, and ConstantDesc. The Constable and ConstantDesc interfaces are part of Java 12 Constants API.
Different Ways to Create String in Java
There are two ways to create string objects in java program.
- Using Double Quotes: A shortcut and special way to create strings. This is the easiest and preferred way to create a String object. The string object is created in the String Pool. For example,
String s1 = "Hello";
- Using new operator: We can also use
new
operator too for creating a string object. The string object is stored in the heap space and doesn’t take advantage of the string pool. For example,String s2 = new String("Hello");
Below image illustrates the process of creating strings using both the above methods.
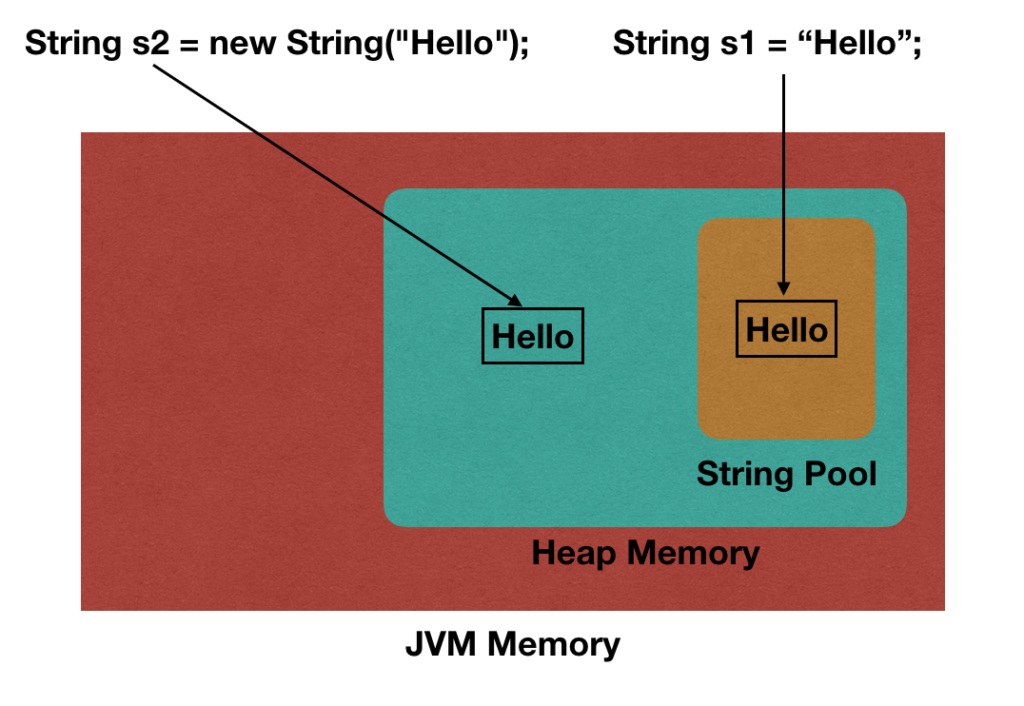
Important Methods of String Class
Let’s look at some of the important methods of String class. Some of these methods are overloaded to handle different scenarios with the arguments.
length()
: Returns the length of the string object.charAt(int index)
: Returns the character value at the given index.toCharArray()
: this method creates a character array from this string.getBytes(String charsetName)
: used to create a byte array from this string.equals(Object anObject)
: used to compare this string with another object.equalsIgnoreCase(String anotherString)
: used to compare this string with another string case-insensitively.compareTo(String anotherString), compareToIgnoreCase(String str)
: compares this string to another string lexicographically. The first one being case-sensitive and the second one performs case insensitive comparison.startsWith(String prefix)
: returns true if this string starts with the given string.endsWith(String suffix)
: returns true if this string ends with the given string.substring(int beginIndex, int endIndex)
: returns a substring of this string.concat(String str)
: Concatenates the given string to the end of this string and return it.replace(char oldChar, char newChar)
: returns a new string after replacing oldChar with the newChar.matches(String regex)
: checks whether this string matches the given regular expression.split(String regex)
: splits this string into a string array using the regular expression argument.join(CharSequence delimiter, CharSequence... elements)
: A utility method to join many strings into a new string with the specified delimiter. We can use this method to create a CSV record from the array of strings.toLowerCase(), toUpperCase()
: used to get the lowercase and uppercase version of this string.trim()
: used to remove leading and trailing whitespaces from this string.strip(), stripLeading(), stripTrailing()
: returns new string after stripping white spaces from this string. If you are confused what’s the difference betweenstrip()
andtrim()
– there are none. Both of them perform the same task butstrip()
method is more readable. It goes in line with similar methods in other programming languages.isBlank()
: returns true if the string is empty or contains only white spaces.lines()
: introduced in Java 11, returns a stream of lines from this string.indent(int n)
: introduced in Java 12, returns an indented string based on the argument value.transform(Function<? super String, ? extends R> f)
: introduced in Java 12 to apply a function to this string. The function should accept a single string argument and return R.format(String format, Object... args)
: returns a formatted string using the specified format and arguments.valueOf(Object obj)
: returns the string representation of the given object. There are overloaded versions to work with primitive data types, arrays, and objects.intern()
: returns the string from the string pool.repeat(int count)
: returns a new string after concatenating this string specified times.describeConstable(), resolveConstantDesc(MethodHandles.Lookup lookup)
: implemented for Java 12 Constants API.
Benefits of String Immutability
- String Pool is possible because String is immutable. Hence saving memory and fast performance.
- More secure since we can’t change the value of string object.
- Thread safety while working with strings in a multi-threaded environment.
- Class loading is more secure since the string value passed as an argument to load a class can’t be changed.
Quick Word on StringBuffer and StringBuilder
String immutability provides a lot of benefits. But, when we have to manipulate strings, it causes a lot of memory and inefficient operations.
That’s why there are two classes for string manipulation – StringBuffer and StringBuilder. Both of them provide similar operations. But, StringBuffer is synchronized and StringBuilder is not.
That’s why there are two classes for string manipulation – StringBuffer and StringBuilder. Both of them provide similar operations. But, StringBuffer is synchronized and StringBuilder is not.
Java String Pool
Java String Pool is the special area in the heap memory to store string objects. There are two ways to create string objects. If we use double quotes to create a string object, it’s stored in the string pool. If we use the new operator to create a string, it’s created in the heap memory.
How Does Java String Pool Works?
- When we create a string literal, it’s stored in the string pool.
- If there is already a string with the same value in the string pool, then new string object is not created. The reference to the existing string object is returned.
- Java String Pool is a cache of string objects. It’s possible because string is immutable.
- If we create a string object using new operator, it’s created in the heap area. If we want to move it to the string pool, we can use
intern()
method. - String Pool is a great example of Flyweight design pattern.
Understanding String Pool With a Program
Let’s understand the working of string pool with a simple program.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
| package net.javastring.strings; public class JavaStringPool { public static void main(String[] args) { String s1 = "Hello" ; String s2 = "Hello" ; String s3 = new String( "Hi" ); String s4 = "Hi" ; System.out.println( "s1 == s2? " + (s1 == s2)); System.out.println( "s3 == s4? " + (s3 == s4)); s3 = s3.intern(); System.out.println( "s3 == s4? " + (s3 == s4)); } } |
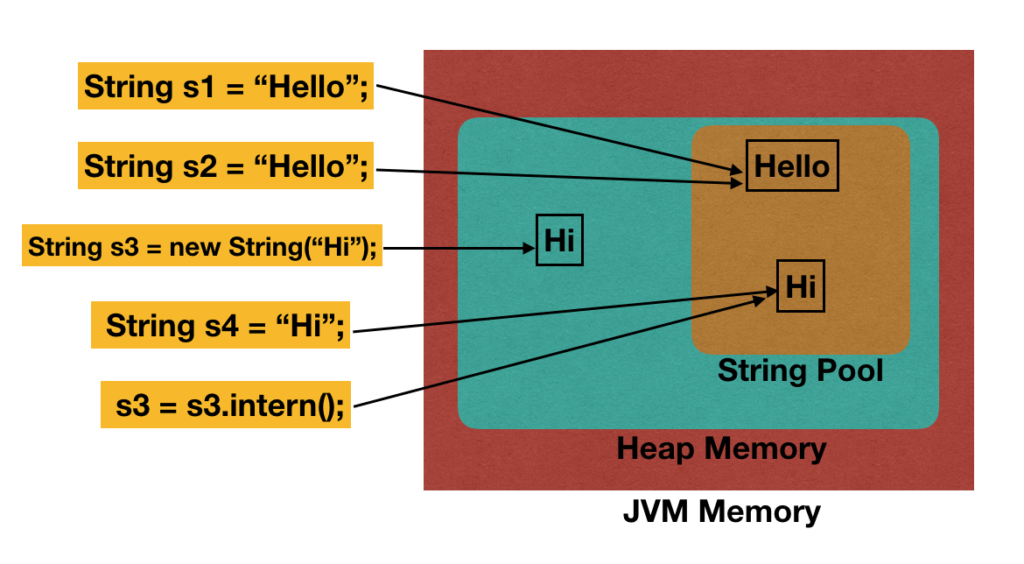
- When we create the first string s1, there is no string with the value “Hello” in the string pool. So a string “Hello” is created in the pool and its reference is assigned to s1.
- When we are creating second string s2, there is already a string with the value “Hello” in the string pool. The existing string reference is assigned to s2.
- When we create third string s3, it’s created in the heap area because we are using the new operator.
- When we create fourth string s4, a new string with the value “Hi” is created in the pool and its reference is assigned to s4.
- When we compare
s1 == s2
, it returnstrue
because both the variables are referring to the same string object. - When we compare
s3 == s4
, it returns false because they point to the different string objects. - When we call
intern()
method on s3, it checks if there is any string in the pool with the value “Hi”? Since we already have a string with this value in the pool, its reference is returned and assigned to s3. - Now
s3 == s4
returnstrue
because both the variables are referring to the same string objects.
What are the Benefits of Java String Pool?
Let’s look at some of the benefits of java string pool.
- Java String Pool allows caching of string objects. This saves a lot of memory for JVM that can be used by other objects.
- Java String Pool helps in better performance of the application because of reusability. It saves time to create a new string if there is already a string present in the pool with the same value.
Why we should never create String using new operator?
Let’s look at the code snippet to create a string using new operator.
String s3 = new String("Hi");
- A new string with value “Hi” is created in the string pool.
- Then the String constructor is called by passing the string created in the above step.
- A new string is created in the heap space and its reference is assigned to s3.
- The string created in the first step is orphan and available for garbage collection.
- We ended up creating two strings when we wanted to create a single string in the heap area.
Java String contains() Method Examples
Java String
contains()
method returns true
if this string contains the given character sequence.Java String contains() method signature
String contains() method signature is:
public boolean contains(CharSequence s)
Important Points for contains() method
- This utility method was introduced in Java 1.5
- It uses
indexOf()
method to check if this string contains the argument string or not. - If the argument is
null
thenNullPointerException
will be thrown. - This method is case sensitive. For example,
"x".contains("X");
will returnfalse
. - You can convert both the strings to lowercase for case insensitive operation. For example,
"x".toLowerCase().contains("X".toLowerCase());
will return true.
Java String equals() Method – Always Use This to Check String Equality
Java String equals() method compares this string to another object.
Java String equals() Signature
String equals() method signature is:
1
| public boolean equals(Object anObject) |
The result is
true
if the argument is String having the same value as this string. For all other cases, it will return false
. If we pass null
argument, then the method will return false
.Why equals() Method Parameter is Not String?
It’s an interesting question. It makes prefect sense to have the method parameter as String because we are checking equality between two string objects.
So why this method argument is Object?
It’s because String equals() method is overriding Object class equals() method. You can confirm this by looking at the equals() method java documentation too.
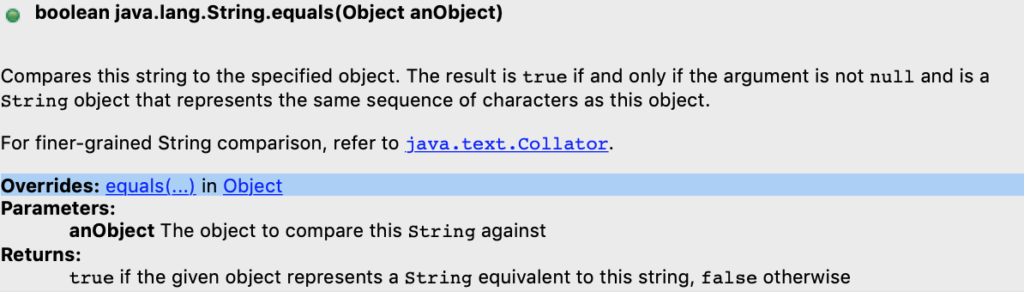
Important Points about String equals() Method
- Java String
equals()
method overrides the Object class equals() method. - If you want to check two strings for equality, you should always use equals() method.
- String equals() method doesn’t throw any exception. It always returns a boolean result.
- If you are looking to check equality ignoring case, then use
equalsIgnoreCase()
method.
Java String equals() Method Example
Let’s look at a simple example of String equals() method.
1
2
3
4
5
6
7
8
9
10
11
12
13
| package net.javastring.strings; public class JavaStringEquals { public static void main(String[] args) { String s1 = "abc" ; String s2 = "abc" ; System.out.println( "s1 is equal to s2? " + (s1.equals(s2))); } } |
Java String equals() and equalsIgnoreCase() Example
Let’s look at another example where we will accept two strings from user input. Then we will check their equality using equals() and equalsIgnoreCase() methods.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
| package net.javastring.strings; import java.util.Scanner; public class JavaStringEquals { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println( "Enter The First String:" ); String str1 = sc.nextLine(); System.out.println( "Enter The Second String:" ); String str2 = sc.nextLine(); sc.close(); System.out.println( "Both the input Strings are Equal? = " + str1.equals(str2)); System.out.println( "Both the input Strings are Case Insensitive Equal? = " + str1.equalsIgnoreCase(str2)); } } |
Output:
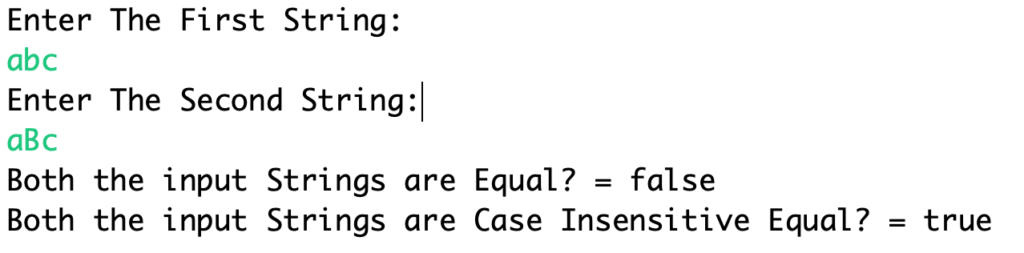
Java String split() Method
Java String split() method returns a String array. It accepts string argument and treats it as regular expression. Then the string is split around the matches found.
String split() Method Syntax
There are two overloaded split() methods.
public String[] split(String regex, int limit)
: The limit argument defines the result string array size. If the limit is 0 or negative then all possible splits are part of the result string array.public String[] split(String regex)
: This is a shortcut method to split the string into maximum possible elements. This method callssplit(regex, 0)
method.
Important Points About String split() Method
- The result string array contains the strings in the order of their appearance in this string.
- If there are no matches, then the result string array contains a single string. The string element has the same value as this string.
- We can use split() method to convert CSV data into a string array.
- Trailing empty strings are not included in the result. For example,
"A1B2C3D4E5".split("[0-9]")
will result in the string array[A, B, C, D, E]
.
Java String split() Examples
Let’s look at some simple examples of split() method.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
| package net.javastring.strings; import java.util.Arrays; public class JavaStringSplit { public static void main(String[] args) { String s = "Hello World 2019" ; String[] words = s.split( " " ); System.out.println(Arrays.toString(words)); String s1 = "A1B2C3D4E5" ; String[] characters = s1.split( "[0-9]" ); System.out.println(Arrays.toString(characters)); String[] twoWordsArray = s.split( " " , 2 ); System.out.println(Arrays.toString(twoWordsArray)); System.out.println(Arrays.toString(s.split( "X" , 2 ))); String s2 = "A1B2C3D " ; System.out.println(Arrays.toString(s2.split( "[0-9]" ))); } } |
Output:
1
2
3
4
5
| [Hello, World, 2019 ] [A, B, C, D, E] [Hello, World 2019 ] [Hello World 2019 ] [A, B, C, D ] |
String split() Examples using JShell
We can run some examples using jshell too.
1
2
3
4
5
6
7
8
9
10
11
12
13
| jshell> "Hello World 2019" .split( " " ); $ 34 ==> String[ 3 ] { "Hello" , "World" , "2019" } jshell> "A1B2C3D4E5" .split( "[0-9]" ); $ 35 ==> String[ 5 ] { "A" , "B" , "C" , "D" , "E" } jshell> "Hello World 2019" .split( " " , 2 ); $ 36 ==> String[ 2 ] { "Hello" , "World 2019" } jshell> " A1B2C3D " .split( "[0-9]" ); $ 37 ==> String[ 4 ] { " A" , "B" , "C" , "D " } jshell> |
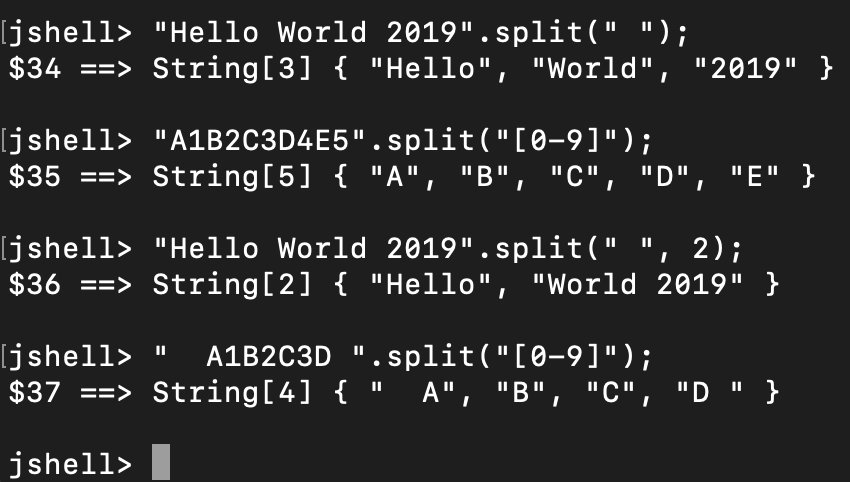
Java String length() Method Examples
Java String length() method returns the length of this string. The output is the number of Unicode code units in the string.
Java String length() Method Example
Let’s look at a simple program for finding the length of a string in Java.
1
2
3
4
5
6
7
8
9
10
| package net.javastring.strings; public class JavaStringLength { public static void main(String args[]) { String s1 = "Hello" ; System.out.println(s1.length()); } } |
Output:
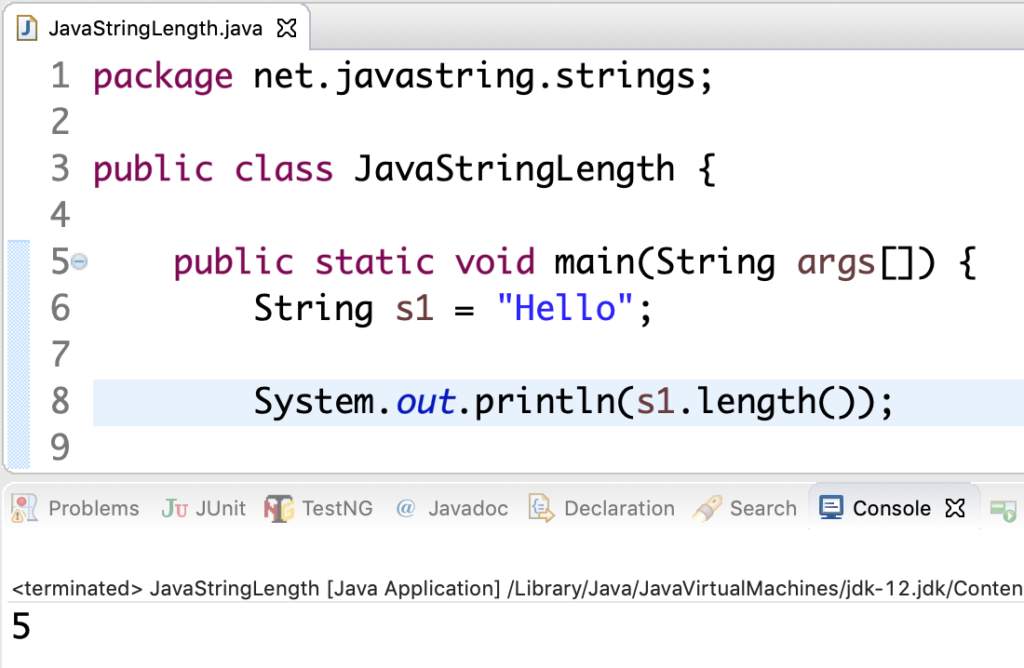
What happens when String has Unicode characters?
We can create a string object with Unicode value too. Let’s look at an example to find the length of a string having Unicode value. We will use JShell for this example.
1
2
3
4
5
| jshell> String s2 = "\u00A9" ; s2 ==> "©" jshell> s2.length(); $ 11 ==> 1 |
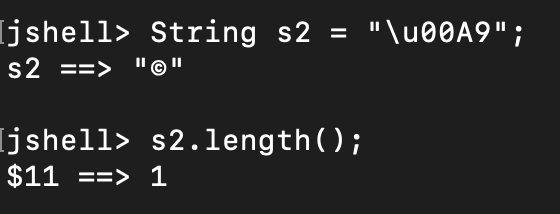
Alternative Ways to Find the Length of String
This is an interesting interview question. You will have to write a program to find the length of the String without using the length() method. Let’s look at two alternative ways to find the length of the string.
1. Using Character Array
We can convert the string to the character array. Then use its length attribute to find the length of the string.
1
2
3
4
5
| String s3 = "Welcome To \u00A9 JavaString.net" ; char [] ca = s3.toCharArray(); System.out.println(ca.length); // 27 |
2. Using String lastIndexOf() method
We can use lastIndexOf() method cleverly to get the length of the string.
1
2
3
4
5
| String s4 = "Hi\nHowdy \u00A9 Java " ; int length = s4.lastIndexOf( "" ); System.out.println(length); // 16 |
Let’s look at the output in JShell. The newline character length is also considered as 1.
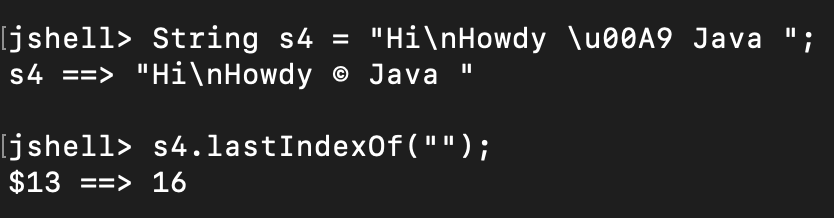
Conclusion
Java String length() method returns the length of the string. The newline character and tab character length is 1. If the string has Unicode value, they are considered as a single character.
We looked into some alternate ways to find the length of the string. But, always use the length() method to get the length of the string.
Important Points for String in Switch Case
- Java switch case with string is more readable than the multiple if-else if-else blocks.
- The switch case matching is case sensitive, so “java” will not match for input string “Java”.
- If the input string is null, switch-case will throw NullPointerException. So have a null check in place before writing the switch-case code.
- The bytecode generated by switch-case is more efficient than the if-else blocks. You can check this in the reference article below.
- You can convert the input string and all the case values to either uppercase or lowercase for case insensitive match.
Java String Comparison – 5 Ways You MUST Know
String comparison is a common operation in programming. We compare two strings to check if they are equal or not. Sometimes, we compare two strings to check which of them comes first lexicographically. This is useful when we have to sort a collection of strings. In this tutorial, we will look at the various ways for string comparison in Java.
String Comparison in Java
We can compare two strings using following ways.
- equals() method
- == operator
- compareTo() method
- compareToIgnoreCase() method
Collator
compare() method
Let’s look into these string comparison methods one by one.
1. equals() method
We can compare two strings for equality using
equals()
method. Java string is case sensitive, so the comparison is also case sensitive. If you want to test equality without case consideration, use equalsIgnoreCase()
method.
1
2
3
4
5
6
7
8
9
10
11
12
13
| jshell> String s1 = "Hello" ; s1 ==> "Hello" jshell> s1.equals( "Hello" ); $ 2 ==> true jshell> s1.equals( "HELLO" ); $ 3 ==> false jshell> s1.equalsIgnoreCase( "HELLO" ); $ 4 ==> true jshell> |
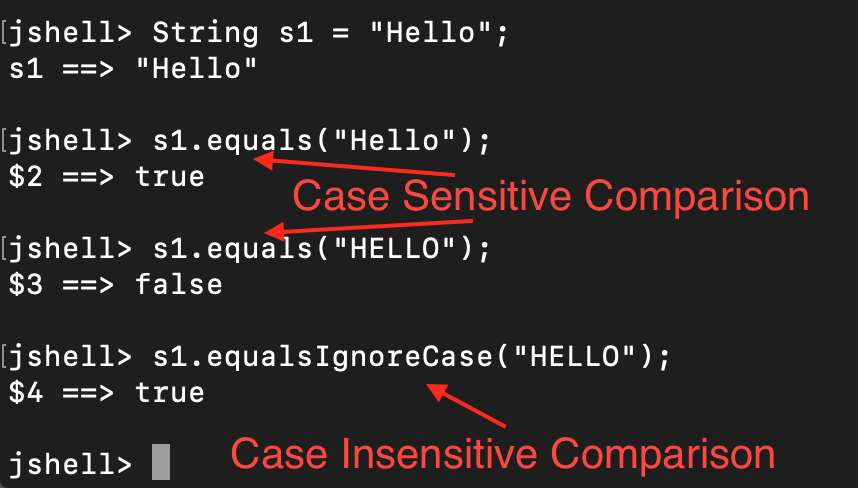
2. == operator
Java == operator returns true if both the variables are referring to the same object. Java String is immutable, so there is no point in using == for checking equality. You should always use the
equals()
method for that.
Let’s look at some examples of using == operator for string comparison.
1
2
3
4
5
6
| String s1 = "Hello" ; String s2 = "Hello" ; String s3 = new String( "Hello" ); System.out.println(s1 == s2); // true System.out.println(s1 == s3); // false |
In the above code snippet, all the string objects have the same value. Strings s1 and s2 are in the pool referring to the same object. String s3 is created in the heap space because of
new
operator. That’s why s1==s2
is true
and s1==s3
is false
.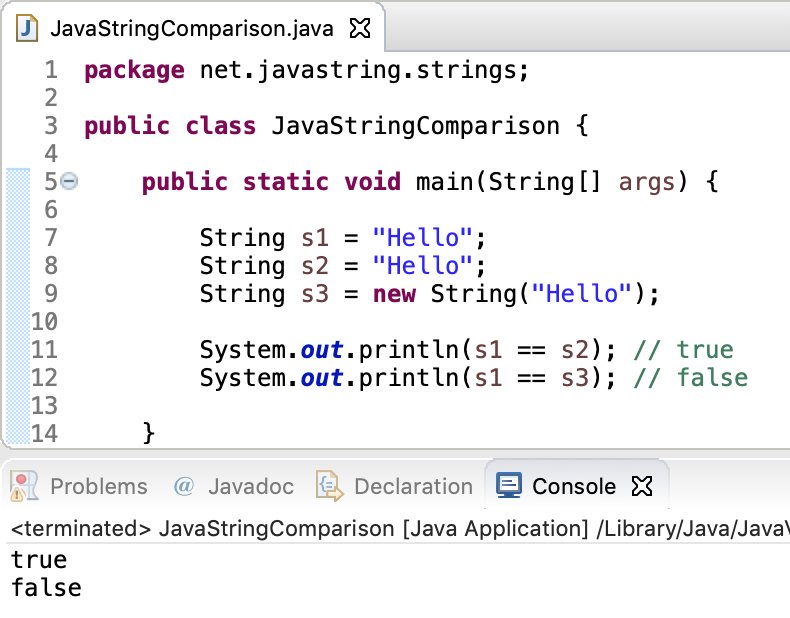
3. compareTo() Method
This method compares the two string lexicographically. The output is always an integer value. It depends on the following comparison rules. String class implements
Comparable
interface. This is the method used when we try to sort an array or collection of strings.- If the string comes before the argument string, the output is negative.
- The characters in both strings are compared one by one.
- If the characters at any index don’t match, then the difference between their code points is the output. So the output will be
this.charAt(n)-argumentString.charAt(n)
if the characters don’t match at index ‘n’. - If the characters match at all the indexes, then the shorter length string precedes the longer length string. So the output is negative. The value is the difference between the lengths of the strings.
- If both the strings have the same value, the output is 0. In this case,
equals()
between these two strings must betrue
.
Let’s look at some examples of compareTo() method and understand the output.
"Hello".compareTo("Hi")
: returns -4 because the characters are different at index 1. Code point of ‘e’ is 101 and the code point of ‘i’ is 105. The difference between them is -4."Hi".compareTo("Hi")
: returns 0 because both the strings are equal."Hi".compareTo("Hii")
: returns -1. The characters are the same in all the indexes of both the strings. The output is negative because “Hi” comes before “Hii”. The output value is 1 because their length difference is 1."Hii".compareTo("Hi")
: returns 1. The output is positive because “Hii” comes after “Hi”. The difference in their length is 1."He".compareTo("Hello")
: returns -3. You can figure out the output using the above logic.- “Hello”.compareTo(“HI”): returns 28. The code point of ‘e’ is 101 and ‘I’ is 73.
4. compareToIgnoreCase() Method
This method works in the same way as compareTo() method. The only difference is that the comparison is case insensitive. The strings are converted to upper case or lower case before the comparison. This is useful when you want to sort a collection of strings without considering their case.
Let’s look at some examples of compareToIgnoreCase() method.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
| package net.javastring.strings; public class JavaStringComparison { public static void main(String[] args) { System.out.println( "H" .compareToIgnoreCase( "h" )); // 0 System.out.println( "HI" .compareToIgnoreCase( "hi" )); // 0 System.out.println( "Hello" .compareTo( "HI" )); // 28 System.out.println( "HI" .codePointAt( 1 )); // 73 System.out.println( "Hello" .compareToIgnoreCase( "HI" )); // -4 } } |
Output:
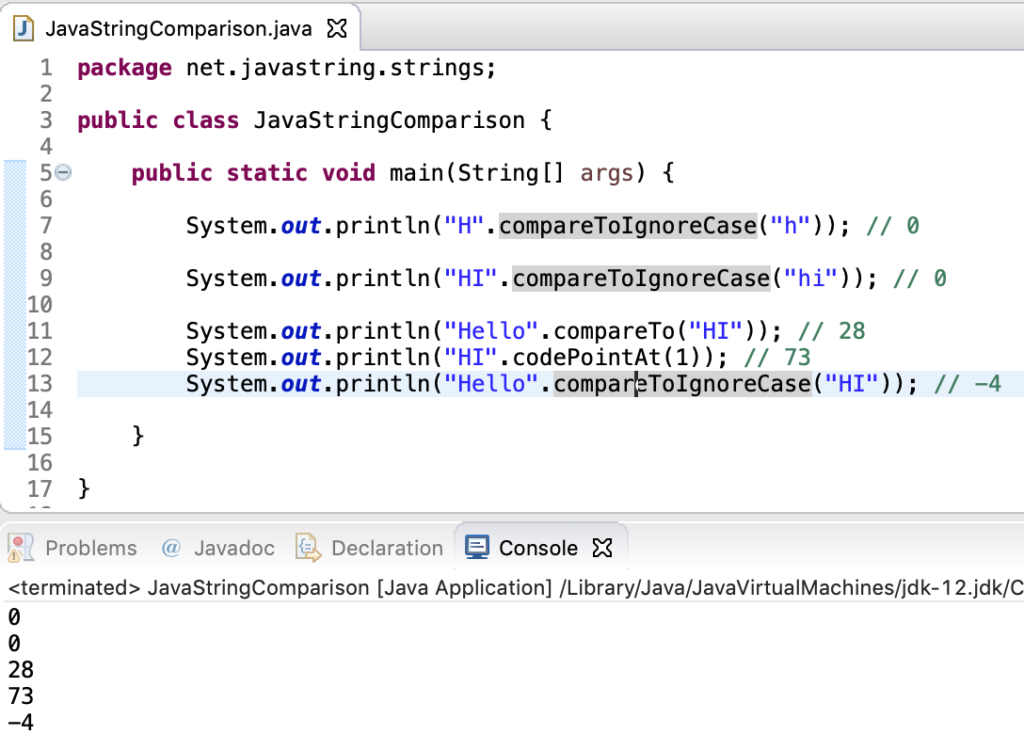
5. Collator class for locale-specific comparison
We can use Collator class for locale-specific string comparison. We can define our own comparison rules to compare the strings. Let’s look at a simple example of using Collator class for locale-specific comparison. We will also check how to define the custom comparison rules.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
| package net.javastring.strings; import java.text.Collator; import java.text.ParseException; import java.text.RuleBasedCollator; import java.util.Locale; public class JavaStringComparisonCollator { public static void main(String[] args) throws ParseException { Collator collator = Collator.getInstance(); Collator collatorDE = Collator.getInstance(Locale.GERMANY); System.out.println(collator.compare( "ABC" , "B" )); // -1 System.out.println(collatorDE.compare( "ABC" , "B" )); // -1 String rules = "< B < A" ; RuleBasedCollator rbc = new RuleBasedCollator(rules); System.out.println(rbc.compare( "ABC" , "B" )); // 1 } } |
Conclusion
There are various ways to compare two strings.
- If you want to check for equality, then use equals() method.
- For lexicographical comparison, use
compareTo()
method. - For case insensitive lexicographically comparison, use
compareToIgnoreCase()
method. - If you want a locale-specific comparison, use
Collator
class. - For specifying your own comparison rules, use
RuleBasedCollator
class.