When to use abstract classes and abstract methods with an example
There are situations in which we will want to define a superclass that declares the structure of a given abstraction without providing a complete implementation of every method. That is, sometimes we will want to create a superclass that only defines a generalization form that will be shared by all of its subclasses, leaving it to each subclass to fill in the details.
Consider a classic “shape” example, perhaps used in a computer-aided design system or game simulation. The base type is “shape” and each shape has a color, size and so on. From this, specific types of shapes are derived(inherited)-circle, square, triangle and so on – each of which may have additional characteristics and behaviors. For example, certain shapes can be flipped. Some behaviors may be different, such as when you want to calculate the area of a shape. The type hierarchy embodies both the similarities and differences between the shapes.
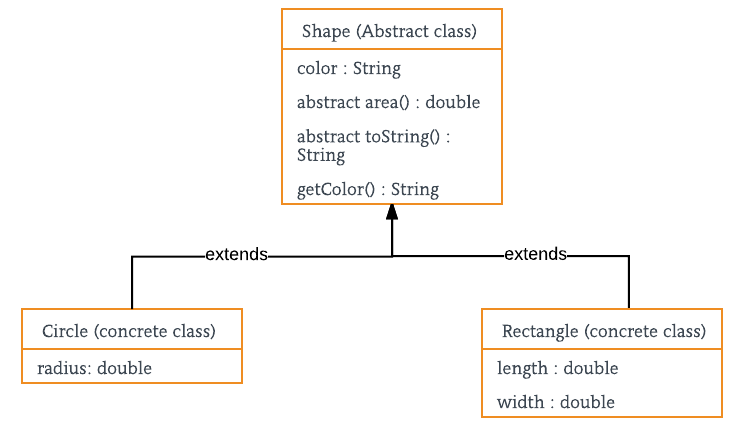
// Java program to illustrate the // concept of Abstraction abstract class Shape { String color; // these are abstract methods abstract double area(); public abstract String toString(); // abstract class can have constructor public Shape(String color) { System.out.println( "Shape constructor called" ); this .color = color; } // this is a concrete method public String getColor() { return color; } } class Circle extends Shape { double radius; public Circle(String color, double radius) { // calling Shape constructor super (color); System.out.println( "Circle constructor called" ); this .radius = radius; } @Override double area() { return Math.PI * Math.pow(radius, 2 ); } @Override public String toString() { return "Circle color is " + super .color + "and area is : " + area(); } } class Rectangle extends Shape{ double length; double width; public Rectangle(String color, double length, double width) { // calling Shape constructor super (color); System.out.println( "Rectangle constructor called" ); this .length = length; this .width = width; } @Override double area() { return length*width; } @Override public String toString() { return "Rectangle color is " + super .color + "and area is : " + area(); } } public class Test { public static void main(String[] args) { Shape s1 = new Circle( "Red" , 2.2 ); Shape s2 = new Rectangle( "Yellow" , 2 , 4 ); System.out.println(s1.toString()); System.out.println(s2.toString()); } } |
Output:
Shape constructor called Circle constructor called Shape constructor called Rectangle constructor called Circle color is Redand area is : 15.205308443374602 Rectangle color is Yellowand area is : 8.0Q-What is interface?
ans:- interface is contract between client and developer.
note:-"interface contain common method prototype that will implemented by multiple subclasses."
real time senario example:-
suppose i have banking application... in banking application i have four module.
(1)saving a/c
(2)deposit a/c
(3)couurent a/c
(4)fixed deposit
so there is chance that all this module will be developed by deffrent-2 developer or diffrent-2 company also there is a chance so what i want...
i want there are some method, some 20 method i have, that 20 methos should be same accors all the module, saving a/c customer or developer also has to override the same methos or other developer also has to override.
implementation may change but if they want to check the balance get the balance methos is there
its take account number as a perameter, return type is duble
withdraw method is there, deposit method is there.
so suppose these method prototype are common amoung multiple sub classe's
note:- if you want to centralized common method prototype you can write inside the interface
so interface contain common method prototype that wil be implemented by multiple sub classe's
Example of Interface
interface Person { void run(); // abstract method } class A implements Person { public void run() { System.out.println("Run fast"); } public static void main(String args[]) { A obj = new A(); obj.run(); } }
Output
Run fast
Multiple Inheritance using interface
Example
interface Developer { void disp(); } interface Manager { void show(); } class Employee implements Developer, Manager { public void disp() { System.out.println("Hello Good Morning"); } public void show() { System.out.println("How are you ?"); } public static void main(String args[]) { Employee obj=new Employee(); obj.disp(); obj.show(); } }
Output
Hello Good Morning How are you ?
Interface is similar to class which is collection of public static final variables (constants) and abstract methods.
The interface is a mechanism to achieve fully abstraction in java. There can be only abstract methods in the interface. It is used to achieve fully abstraction and multiple inheritance in Java.
Why we use Interface ?
- It is used to achieve fully abstraction.
- By using Interface, you can achieve multiple inheritance in java.
- It can be used to achieve loose coupling.
properties of Interface
- It is implicitly abstract. So we no need to use the abstract keyword when declaring an interface.
- Each method in an interface is also implicitly abstract, so the abstract keyword is not needed.
- Methods in an interface are implicitly public.
- All the data members of interface are implicitly public static final.
When we use abstract and when Interface
If we do not know about any things about implementation just we have requirement specification then we should be go for Interface
If we are talking about implementation but not completely (partially implemented) then we should be go for abstract
Rules for implementation interface
- A class can implement more than one interface at a time.
- A class can extend only one class, but implement many interfaces.
- An interface can extend another interface, similarly to the way that a class can extend another class.
Q-What is Abstract class in Java?
A class that is declared as abstract is known as abstract class.
Syntax:
abstract class <class-name>{}
An abstract class is something which is incomplete and you cannot create instance of abstract class.
If you want to use it you need to make it complete or concrete by extending it.
A class is called concrete if it does not contain any abstract method and implements all abstract method inherited from abstract class or interface it has implemented or extended.
Q-What is Abstract class in Java?
A class that is declared as abstract is known as abstract class.Syntax:
abstract class <class-name>{}
An abstract class is something which is incomplete and you cannot create instance of abstract class.
If you want to use it you need to make it complete or concrete by extending it.
Why interface have no constructor ?
Because, constructor are used for eliminate the default values by user defined values, but in case of interface all the data members are public static final that means all are constant so no need to eliminate these values.
Other reason because constructor is like a method and it is concrete method and interface does not have concrete method it have only abstract methods that's why interface have no constructor.
Java Interface with example:-
Relationship between class and Interface
- Any class can extends another class
- Any Interface can extends another Interface.
- Any class can Implements another Interface
- Any Interface can not extend or Implements any class.
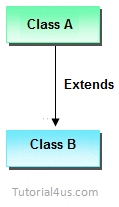
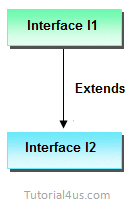
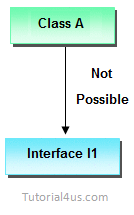
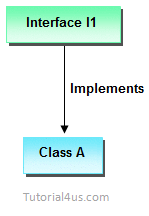
Difference between Abstract class and Interface
Abstract class | Interface | |
---|---|---|
1 | It is collection of abstract method and concrete methods. | It is collection of abstract method. |
2 | There properties can be reused commonly in a specific application. | There properties commonly usable in any application of java environment. |
3 | It does not support multiple inheritance. | It support multiple inheritance. |
4 | Abstract class is preceded by abstract keyword. | It is preceded by Interface keyword. |
5 | Which may contain either variable or constants. | Which should contains only constants. |
6 | The default access specifier of abstract class methods are default. | There default access specifier of interface method are public. |
7 | These class properties can be reused in other class using extend keyword. | These properties can be reused in any other class using implements keyword. |
8 | Inside abstract class we can take constructor. | Inside interface we can not take any constructor. |
9 | For the abstract class there is no restriction like initialization of variable at the time of variable declaration. | For the interface it should be compulsory to initialization of variable at the time of variable declaration. |
10 | There are no any restriction for abstract class variable. | For the interface variable can not declare variable as private, protected, transient, volatile. |
11 | There are no any restriction for abstract class method modifier that means we can use any modifiers. | For the interface method can not declare method as strictfp, protected, static, native, private, final, synchronized. |
1:Interfaces should contain only abstract methods
2:-nterfaces are implemented by the class using ‘implements‘ keyword.
3:-By default, Every field of an interface is public, static and final,
You can’t change the value of a field once they are initialized. Because they are static and final.
4:-By default, All methods of an interface are public and abstract.
5:-While implementing any interface methods inside a class, that method must be declared as public. Because, according to method overriding rule, you can’t reduce visibility of super class method.
6:-As we all know that, any class in java can not extend more than one class. But class can implement more than one interfaces
- /*
- Java Interface example.
- This Java Interface example describes how interface is defined and
- being used in Java language.
- Syntax of defining java interface is,
- <modifier> interface <interface-name>{
- //members and methods()
- }
- */
- //declare an interface
- interface IntExample{
- /*
- Syntax to declare method in java interface is,
- <modifier> <return-type> methodName(<optional-parameters>);
- IMPORTANT : Methods declared in the interface are implicitly public and abstract.
- */
- public void sayHello();
- }
- }
- /*
- Classes are extended while interfaces are implemented.
- To implement an interface use implements keyword.
- IMPORTANT : A class can extend only one other class, while it
- can implement n number of interfaces.
- */
- public class JavaInterfaceExample implements IntExample{
- /*
- We have to define the method declared in implemented interface,
- or else we have to declare the implementing class as abstract class.
- */
- public void sayHello(){
- System.out.println("Hello Visitor !");
- }
- public static void main(String args[]){
- //create object of the class
- JavaInterfaceExample javaInterfaceExample = new JavaInterfaceExample();
- //invoke sayHello(), declared in IntExample interface.
- javaInterfaceExample.sayHello();
- }
- }
- /*
- OUTPUT of the above given Java Interface example would be :
- Hello Visitor !
- */
abstract class:-
abstract class that is having paritial implementation is called abstract class.
now in abstract class you can write abstract methos also concrete method now why to write concrete method.
there are some situation where multiple suclasses have some method behaviour is common
suppose print statement method is there its responsibility to take the account number and print the statement
suppose this method behaviour is common in all the subclass so why to write the same method body in all the subclasses it will give code duplication problem
note:-in future if you want to change all the subclass's you have to modify so better what we can do
we can write that common method behaviour in the abstract class
note:-so we can say abstract class contain common methos behaviour
and concreate contaion specifig method behaviour