Providing solution(interview tips) and doing implementation based on Liferay Portal and Java/J2EE technologies.
Sunday 31 December 2017
Friday 29 December 2017
Git (version control)
Q-What is Git ??
Ans- Git is a Distributed Version Control tool that supports distributed non-linear workflows by providing data assurance for developing quality software.
Generally We have two type of version control system
1-Centralized Version Control System (CVCS(SVN-Subversion)).
2-Distributed Version Control System (DVCS(Git)).
1-Centralized VCS(SubVersion)
Centralized version control system (CVCS) uses a central server to store all files and enables team collaboration. It works on a single repository to which users can directly access a central server.
Note- Here repository is new word---Repository is place where you will have all your code.
Ans- Git is a Distributed Version Control tool that supports distributed non-linear workflows by providing data assurance for developing quality software.
The motive of Git is to manage a project or a set of files as they change over time. Git stores this information in a data structure called a Git repository. The repository is the core of Git.
To be very clear, a Git repository is the directory where all of your project files and the related metadata resides.
Generally We have two type of version control system
1-Centralized Version Control System (CVCS(SVN-Subversion)).
2-Distributed Version Control System (DVCS(Git)).
1-Centralized VCS(SubVersion)
Centralized version control system (CVCS) uses a central server to store all files and enables team collaboration. It works on a single repository to which users can directly access a central server.
Note- Here repository is new word---Repository is place where you will have all your code.

The repository in the above diagram indicates a central server that could be local or remote which is directly connected to each of the programmer’s workstation.
Every programmer can extract or update their workstations with the data present in the repository or can make changes to the data or commit in the repository. Every operation is performed directly on the repository.
it has some major drawbacks. Some of them are:
- It is not locally available; meaning you always need to be connected to a network to perform any action.
- Since everything is centralized, in any case of the central server getting crashed or corrupted will result in losing the entire data of the project.
Distributed VCS
These systems do not necessarily rely on a central server to store all the versions of a project file.
In Distributed VCS, every contributor has a local copy or “clone” of the main repository i.e. everyone maintains a local repository of their own which contains all the files and metadata present in the main repository.
You will understand it better by referring to the diagram below:
Advantage suppose your server fails its fine you have local copy
so every machine every developer have own copy so this called distrributed
In this digram we are distrubting repository

As you can see in the above diagram, every programmer maintains a local repository on its own, which is actually the copy or clone of the central repository on their hard drive. They can commit and update their local repository without any interference.
They can update their local repositories with new data from the central server by an operation called “pull” and affect changes to the main repository by an operation called “push” from their local repository.
The act of cloning an entire repository into your workstation to get a local repository gives you the following advantages:
- All operations (except push & pull) are very fast because the tool only needs to access the hard drive, not a remote server. Hence, you do not always need an internet connection.
- Committing new change-sets can be done locally without manipulating the data on the main repository. Once you have a group of change-sets ready, you can push them all at once.
- Since every contributor has a full copy of the project repository, they can share changes with one another if they want to get some feedback before affecting changes in the main repository.
- If the central server gets crashed at any point of time, the lost data can be easily recovered from any one of the contributor’s local repositories.
After knowing Distributed VCS, its time we take a dive into what is Git.
Better how? - Better to manage your code.
How to manage? - Helps maintaining and keeping track of different versions of your code.
Versions?- When you code, you tend to keep adding let’s say modules, maybe bug fixes etc. basically meaning changing your initial code. But sometimes, new addition do not work and you want to go back to the state where it worked.
In such cases, tools like Git comes in handy, because it had been maintaining your changes inn code and preserving every version. So, you can revert back anytime.
How does Git identify changes?- So, every time you make a change in your code, you need to “commit”. Each commit gives you a snapshot of your code.
Where does Git store all these changes?- In a repository. Repository can be thought of a data space where you can store all your files related to your project.
Mostly, you work with two repositories - local, which resides in your system and remote/central which mostly lies on a remote machine - something like GitHub.
Ah! Here comes GitHub!
Technical definition - It is a website where you can host your repository and share them with people.
Friendly definition - It is a Facebook for programmers. Instead of pictures and
Differences between GIt vs SVN:-
The key difference is that it is decentralized. Imagine you are a developer on the road, you develop on your laptop and you want to have source control so that you can go back 3 hours.
With Subversion, you have a Problem: The SVN Repository may be in a location you can't reach (in your company, and you don't have internet at the moment), you cannot commit. If you want to make a copy of your code, you have to literally copy/paste it.
Sunday 17 December 2017
Java and liferay Spring MVC with annotation
Q-What is spring mvc?
ans-Spring MVC is design pattern which provide seperation of presentation layer and business layer
It provides solution to layer an application by separating three concerns business, presentation and control flow. Model contains business logic, controller takes care of the interaction between view and model. Controller gets input from view and coverts it in preferable format for the model and passes to it. Then gets the response and forwards to view. View contains the presentation part of the application.
Spring MVC Advantage:-
1-reusing component
2-Modifying in one layer will not effect other layer.

In Spring MVC we have presentation layer,controller layer,service layer and model layer.
Suppose from presentation layer we have return suppose login.jsp, in
When we click on submit button than one url will be found with that url request will be forward to the server,
-on server in web.xml you have configured dispatcherServlet here dispatcherServlet is the frontController for spring mvc.
DispatcherServlet test client request, it will take url pattern, Using URL Pattern it will contact one component of spring mvc called handlerMapping.
handlerMapping is responsible to take URL, based on that url it will identify which controller class of your application, which method is responsible to process url pattern, so that controller class information and method releted information will send back to the dispatcher.
Finally controller class that particular method will be called, in that method if you use service class that object will be created, it will contact database model layer.
From controller class you returning some string that is viewlogical name that will come to dispatcherServlet.
again dispatcherServlet will give view logical name to the viewResolver, viewResolver is responsible to take string , it will add prefix and suffice
view will be render to the client, view will be return to dispatcherServlet and it will display to client
Liferay Spring MVC Annotation:
1-@RequestMapping("VIEW")
2-@RenderMapping(params = "action=saveRecord")
3-@ResourceMapping(value = "getStateCountry")
ans-Spring MVC is design pattern which provide seperation of presentation layer and business layer
It provides solution to layer an application by separating three concerns business, presentation and control flow. Model contains business logic, controller takes care of the interaction between view and model. Controller gets input from view and coverts it in preferable format for the model and passes to it. Then gets the response and forwards to view. View contains the presentation part of the application.
- The model is contract between controller and view, Model represents data of the application , it contain service layer and data acccess alyer also it has setter and getter method basically model is simple java class which generates dao and dto classes
- The view is responsible for particular jsp page and html page what you displaying on UI ...visualization of your model.
- The controller manages complete lifecycle of application, it is responsible for recieving the request from user and calling backend servicess and makes calls into business objects.
DispatcherServlet
Purpose of dispatcher is recieve the request & map those request to the correct resource such as controller, model & views
DispatcherServlet is the class that manage the entire request handling process.
DispatcherServlet is the class that manage the entire request handling process.
Spring MVC Advantage:-
1-reusing component
2-Modifying in one layer will not effect other layer.
Spring MVC 3.0.0 Execution Flow:-
1-The DispatcherServlet first receives the request.
2-The DispatcherServlet consults the HandlerMapping and invokes the Controller associated with the request.
3-The Controller process the request by calling the appropriate service methods and returns a ModeAndView object to the DispatcherServlet. The ModeAndView object contains the model data and the view name.
4-The DispatcherServlet sends the view name to a ViewResolver to find the actual View to invoke.
5-Now the DispatcherServlet will pass the model object to the View to render the result.
6-The View with the help of the model data will render the result back to the user.
2-The DispatcherServlet consults the HandlerMapping and invokes the Controller associated with the request.
3-The Controller process the request by calling the appropriate service methods and returns a ModeAndView object to the DispatcherServlet. The ModeAndView object contains the model data and the view name.
4-The DispatcherServlet sends the view name to a ViewResolver to find the actual View to invoke.
5-Now the DispatcherServlet will pass the model object to the View to render the result.
6-The View with the help of the model data will render the result back to the user.
Suppose from presentation layer we have return suppose login.jsp, in
When we click on submit button than one url will be found with that url request will be forward to the server,
-on server in web.xml you have configured dispatcherServlet here dispatcherServlet is the frontController for spring mvc.
DispatcherServlet test client request, it will take url pattern, Using URL Pattern it will contact one component of spring mvc called handlerMapping.
handlerMapping is responsible to take URL, based on that url it will identify which controller class of your application, which method is responsible to process url pattern, so that controller class information and method releted information will send back to the dispatcher.
Finally controller class that particular method will be called, in that method if you use service class that object will be created, it will contact database model layer.
From controller class you returning some string that is viewlogical name that will come to dispatcherServlet.
again dispatcherServlet will give view logical name to the viewResolver, viewResolver is responsible to take string , it will add prefix and suffice
view will be render to the client, view will be return to dispatcherServlet and it will display to client
Liferay Spring MVC Annotation:
1-@RequestMapping("VIEW")
2-@RenderMapping(params = "action=saveRecord")
3-@ResourceMapping(value = "getStateCountry")
interview question of Exception Handling in Java
Q- What is exception??
Ans-Exception is abnormal condition which occured at runtime
abnormal means- it will not maintain normal flow.
We use exception to print a user friendly warning message to suer......
we can handle exception:-
1-try
2-catch
3-finally
4-throw
5-throws
Q- Why we use exception handling??
Ans-Here abnormal termination will happen..
Suppose 1000 line code is there inside method...
From 1000 line of code First 900 line code executed successfully and
900 to 905 there 5 line given some problem, if some problem occure in you code,
Than control will transfer to jvm, JVM will analyze the problem, and it will create some exception related object, it will throw that object in your program, if you are not handling object
what will happen JVM will propogate that object to default exception handler
default excption handler will print error message and you program will terminated.
So because of 5 line, I dont want terminate entire program bcoz of 5 line i can use try and catch block
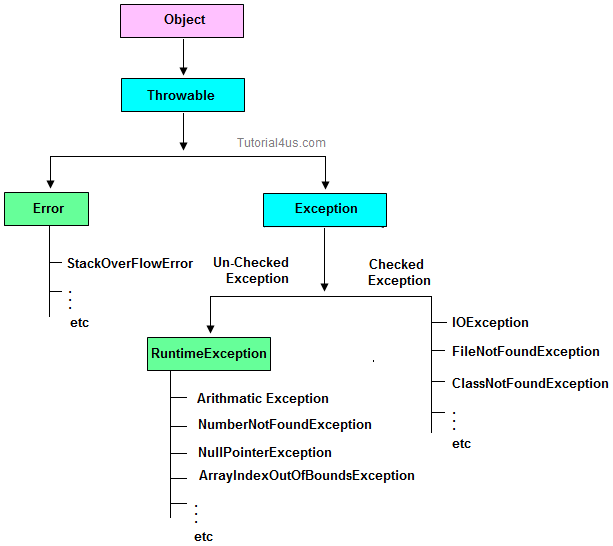
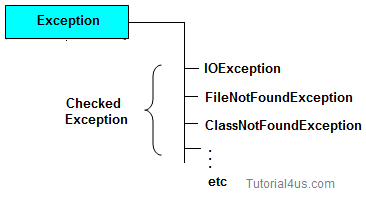
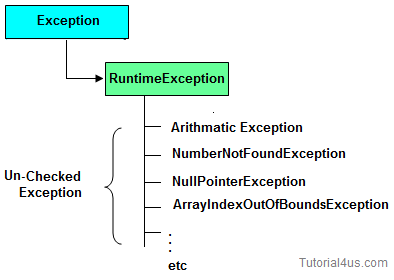
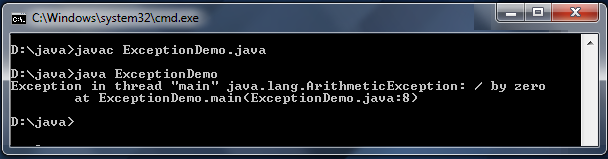
Ans-Exception is abnormal condition which occured at runtime
abnormal means- it will not maintain normal flow.
We use exception to print a user friendly warning message to suer......
we can handle exception:-
1-try
2-catch
3-finally
4-throw
5-throws
Q- Why we use exception handling??
Ans-Here abnormal termination will happen..
Suppose 1000 line code is there inside method...
From 1000 line of code First 900 line code executed successfully and
900 to 905 there 5 line given some problem, if some problem occure in you code,
Than control will transfer to jvm, JVM will analyze the problem, and it will create some exception related object, it will throw that object in your program, if you are not handling object
what will happen JVM will propogate that object to default exception handler
default excption handler will print error message and you program will terminated.
So because of 5 line, I dont want terminate entire program bcoz of 5 line i can use try and catch block
Hierarchy of Exception classes
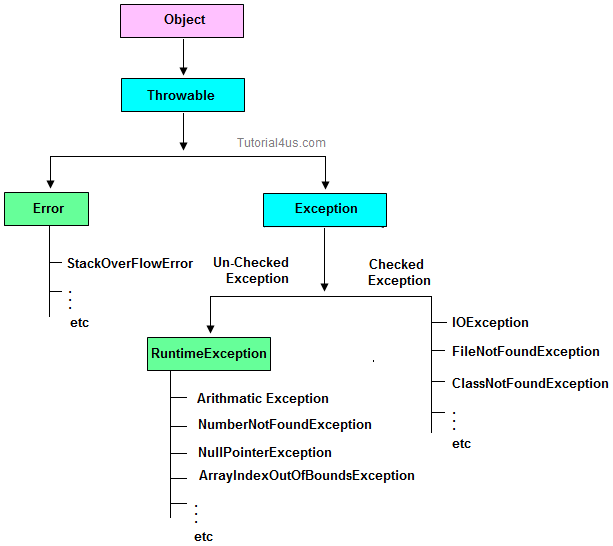
Type of Exception
- Checked Exception
- Un-Checked Exception
Checked Exception
Checked Exception-"if you code inside the method or constructor throwing some exception and if that exception is checked by compiler, compiler is forcing you to report about the exception by writting by try and catch block or by using throws keyword is called checked exception".
are the exception which checked at compile-time. These exception are directly sub-class of java.lang.Exception class.
Only for remember: Checked means checked by compiler so checked exception are checked at compile-time.
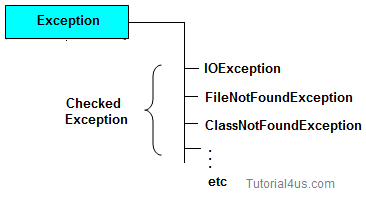
Un-Checked Exception
Un-Checked Exception-means this is aslo called runtime Exception, if some code inside the method throwing exception and compiler is not telling anything wheather exception you want to report or dont report, compiler is not informing anything is called unchecked exception.
are the exception both identifies or raised at run time. These exception are directly sub-class of java.lang.RuntimeException class.
Note: In real time application mostly we can handle un-checked exception.
Only for remember: Un-checked means not checked by compiler so un-checked exception are checked at run-time not compile time.
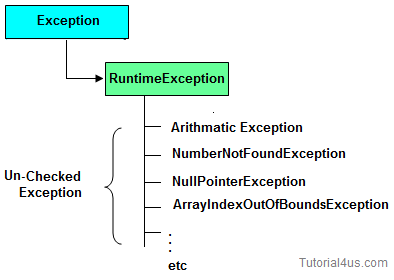
Difference between checked Exception and un-checked Exception
Checked Exception | Un-Checked Exception | |
---|---|---|
1 | checked Exception are checked at compile time | un-checked Exception are checked at run time |
3 | e.g. FileNotFoundException, NumberNotFoundException etc. | e.g. ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. |
Use Five keywords for Handling the Exception
- try
- catch
- finally
- throws
- throw
Syntax for handling the exception
Syntax
try { // statements causes problem at run time } catch(type of exception-1 object-1) { // statements provides user friendly error message } catch(type of exception-2 object-2) { // statements provides user friendly error message } finally { // statements which will execute compulsory }
Example without Exception Handling
Syntax
class ExceptionDemo { public static void main(String[] args) { int a=10, ans=0; ans=a/0; System.out.println("Denominator not be zero"); } }
Abnormally terminate program and give a message like below, this error message is not understandable by user so we convert this error message into user friendly error message, like "denominator not be zero".
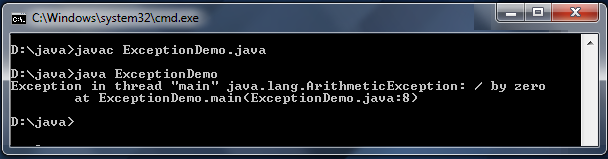
Example of Exception Handling
Example
class ExceptionDemo { public static void main(String[] args) { int a=10, ans=0; try { ans=a/0; } catch (Exception e) { System.out.println("Denominator not be zero"); } } }
Custom Exception in Java
If any exception is design by the user known as user defined or Custom Exception. Custom Exception is created by user.
Rules to design user defined Exception
- Create a package with valid user defined name.
- Create any user defined class.
- Make that user defined class as derived class of Exception or RuntimeException class.
- Declare parametrized constructor with string variable.
- call super class constructor by passing string variable within the derived class constructor.
- Save the program with public class name.java
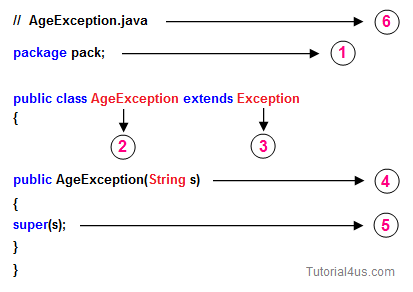
Example
// save by AgeException.java
package nage;
public class AgeException extends Exception
{
public AgeException(String s)
{
super(s);
}
}
// save by AgeException.java package nage; public class AgeException extends Exception { public AgeException(String s) { super(s); } }
Compile: javac -d . AgeException.java
Example
// save by CheckAge.java
package nage;
public class CheckAge
{
public void verify(int age)throws AgeException
{
if (age>0)
{
System.err.print("valid age");
}
else
{
AgeException ae=new AgeException("Invalid age");
throw(ae);
}
}
}
// save by CheckAge.java package nage; public class CheckAge { public void verify(int age)throws AgeException { if (age>0) { System.err.print("valid age"); } else { AgeException ae=new AgeException("Invalid age"); throw(ae); } } }
Compile: javac -d . CheckAge.java
Example
// save by VerifyAgeException
import nage.AgeException;
import nage.CheckAge;
import java.util.*;
public class VerifyAgeException
{
public static void main(String args[])
{
int a;
System.out.println("Enter your age");
Scanner s=new Scanner(System.in);
a=s.nextInt();
try
{
CheckAge ca=new CheckAge();
ca.verify(a);
}
catch(AgeException ae)
{
System.err.println("Age should not be -ve");
}
catch(Exception e)
{
System.err.println(e);
}
}
}
// save by VerifyAgeException import nage.AgeException; import nage.CheckAge; import java.util.*; public class VerifyAgeException { public static void main(String args[]) { int a; System.out.println("Enter your age"); Scanner s=new Scanner(System.in); a=s.nextInt(); try { CheckAge ca=new CheckAge(); ca.verify(a); } catch(AgeException ae) { System.err.println("Age should not be -ve"); } catch(Exception e) { System.err.println(e); } } }
Compile: javac VerifyAgeException.java
finally Block in Exception Handling
Inside finallyblock we write the block of statements which will relinquish (released or close or terminate) the resource (file or database) where data store permanently.
finally block important points
- Finally block will execute compulsory
- Writing finally block is optional.
- You can write finally block for the entire java program
- In some of the circumstances one can also write try and catch block in finally block.
Example
class ExceptionDemo { public static void main(String[] args) { int a=10, ans=0; try { ans=a/0; } catch (Exception e) { System.out.println("Denominator not be zero"); } finally { System.out.println("I am from finally block"); } } }
Output
Denominator not be zero I am from finally block
Difference Between Throw and Throws Keyword
throw
throw is a keyword in java language which is used to throw any user defined exception to the same signature of method in which the exception is raised.Note: throw keyword always should exist within method body.whenever method body contain throw keyword than the call method should be followed by throws keyword.Syntax
class className { returntype method(...) throws Exception_class { throw(Exception obj) } }throws
throws is a keyword in java language which is used to throw the exception which is raised in the called method to it's calling method throws keyword always followed by method signature.Example
returnType methodName(parameter)throws Exception_class.... { ..... }
Subscribe to:
Posts (Atom)