Q- What is exception??
Ans-Exception is abnormal condition which occured at runtime
abnormal means- it will not maintain normal flow.
We use exception to print a user friendly warning message to suer......
we can handle exception:-
1-try
2-catch
3-finally
4-throw
5-throws
Q- Why we use exception handling??
Ans-Here abnormal termination will happen..
Suppose 1000 line code is there inside method...
From 1000 line of code First 900 line code executed successfully and
900 to 905 there 5 line given some problem, if some problem occure in you code,
Than control will transfer to jvm, JVM will analyze the problem, and it will create some exception related object, it will throw that object in your program, if you are not handling object
what will happen JVM will propogate that object to default exception handler
default excption handler will print error message and you program will terminated.
So because of 5 line, I dont want terminate entire program bcoz of 5 line i can use try and catch block
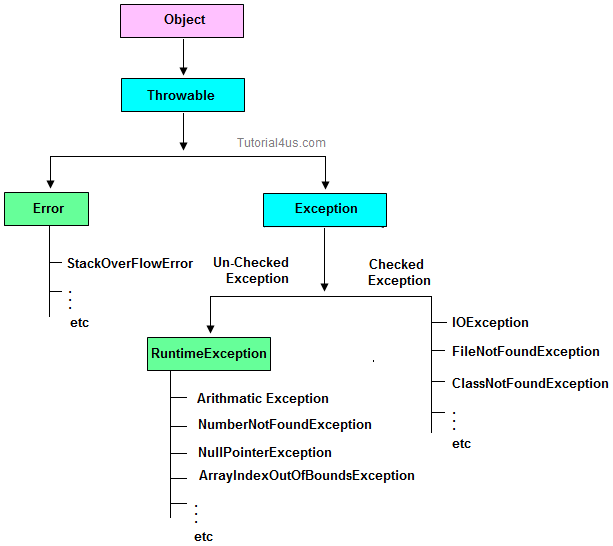
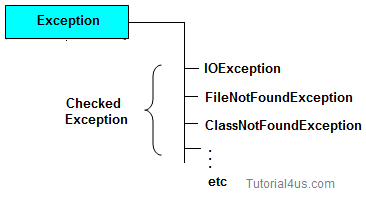
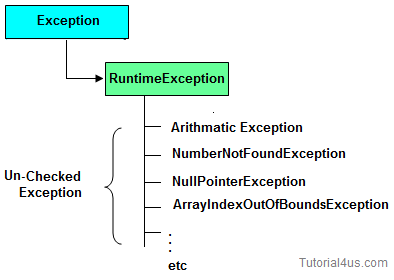
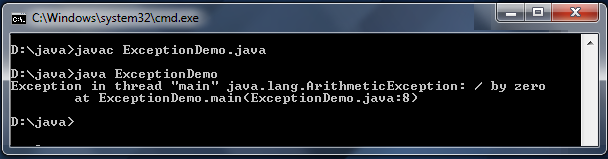
Ans-Exception is abnormal condition which occured at runtime
abnormal means- it will not maintain normal flow.
We use exception to print a user friendly warning message to suer......
we can handle exception:-
1-try
2-catch
3-finally
4-throw
5-throws
Q- Why we use exception handling??
Ans-Here abnormal termination will happen..
Suppose 1000 line code is there inside method...
From 1000 line of code First 900 line code executed successfully and
900 to 905 there 5 line given some problem, if some problem occure in you code,
Than control will transfer to jvm, JVM will analyze the problem, and it will create some exception related object, it will throw that object in your program, if you are not handling object
what will happen JVM will propogate that object to default exception handler
default excption handler will print error message and you program will terminated.
So because of 5 line, I dont want terminate entire program bcoz of 5 line i can use try and catch block
Hierarchy of Exception classes
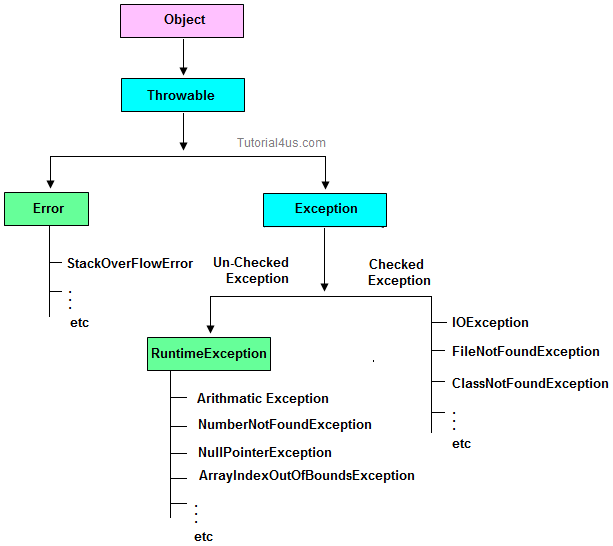
Type of Exception
- Checked Exception
- Un-Checked Exception
Checked Exception
Checked Exception-"if you code inside the method or constructor throwing some exception and if that exception is checked by compiler, compiler is forcing you to report about the exception by writting by try and catch block or by using throws keyword is called checked exception".
are the exception which checked at compile-time. These exception are directly sub-class of java.lang.Exception class.
Only for remember: Checked means checked by compiler so checked exception are checked at compile-time.
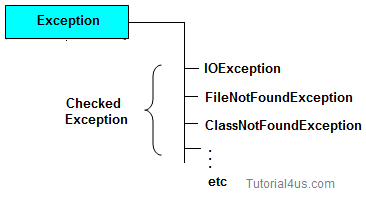
Un-Checked Exception
Un-Checked Exception-means this is aslo called runtime Exception, if some code inside the method throwing exception and compiler is not telling anything wheather exception you want to report or dont report, compiler is not informing anything is called unchecked exception.
are the exception both identifies or raised at run time. These exception are directly sub-class of java.lang.RuntimeException class.
Note: In real time application mostly we can handle un-checked exception.
Only for remember: Un-checked means not checked by compiler so un-checked exception are checked at run-time not compile time.
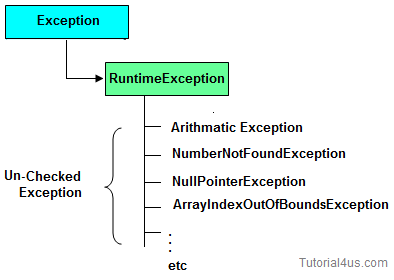
Difference between checked Exception and un-checked Exception
Checked Exception | Un-Checked Exception | |
---|---|---|
1 | checked Exception are checked at compile time | un-checked Exception are checked at run time |
3 | e.g. FileNotFoundException, NumberNotFoundException etc. | e.g. ArithmeticException, NullPointerException, ArrayIndexOutOfBoundsException etc. |
Use Five keywords for Handling the Exception
- try
- catch
- finally
- throws
- throw
Syntax for handling the exception
Syntax
try { // statements causes problem at run time } catch(type of exception-1 object-1) { // statements provides user friendly error message } catch(type of exception-2 object-2) { // statements provides user friendly error message } finally { // statements which will execute compulsory }
Example without Exception Handling
Syntax
class ExceptionDemo { public static void main(String[] args) { int a=10, ans=0; ans=a/0; System.out.println("Denominator not be zero"); } }
Abnormally terminate program and give a message like below, this error message is not understandable by user so we convert this error message into user friendly error message, like "denominator not be zero".
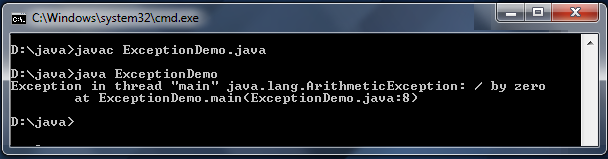
Example of Exception Handling
Example
class ExceptionDemo { public static void main(String[] args) { int a=10, ans=0; try { ans=a/0; } catch (Exception e) { System.out.println("Denominator not be zero"); } } }
Custom Exception in Java
If any exception is design by the user known as user defined or Custom Exception. Custom Exception is created by user.
Rules to design user defined Exception
- Create a package with valid user defined name.
- Create any user defined class.
- Make that user defined class as derived class of Exception or RuntimeException class.
- Declare parametrized constructor with string variable.
- call super class constructor by passing string variable within the derived class constructor.
- Save the program with public class name.java
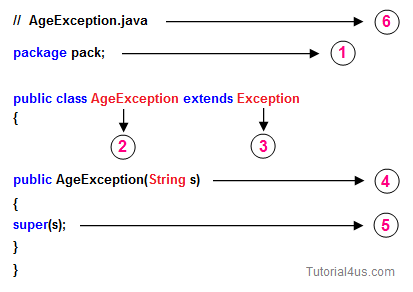
Example
// save by AgeException.java
package nage;
public class AgeException extends Exception
{
public AgeException(String s)
{
super(s);
}
}
// save by AgeException.java package nage; public class AgeException extends Exception { public AgeException(String s) { super(s); } }
Compile: javac -d . AgeException.java
Example
// save by CheckAge.java
package nage;
public class CheckAge
{
public void verify(int age)throws AgeException
{
if (age>0)
{
System.err.print("valid age");
}
else
{
AgeException ae=new AgeException("Invalid age");
throw(ae);
}
}
}
// save by CheckAge.java package nage; public class CheckAge { public void verify(int age)throws AgeException { if (age>0) { System.err.print("valid age"); } else { AgeException ae=new AgeException("Invalid age"); throw(ae); } } }
Compile: javac -d . CheckAge.java
Example
// save by VerifyAgeException
import nage.AgeException;
import nage.CheckAge;
import java.util.*;
public class VerifyAgeException
{
public static void main(String args[])
{
int a;
System.out.println("Enter your age");
Scanner s=new Scanner(System.in);
a=s.nextInt();
try
{
CheckAge ca=new CheckAge();
ca.verify(a);
}
catch(AgeException ae)
{
System.err.println("Age should not be -ve");
}
catch(Exception e)
{
System.err.println(e);
}
}
}
// save by VerifyAgeException import nage.AgeException; import nage.CheckAge; import java.util.*; public class VerifyAgeException { public static void main(String args[]) { int a; System.out.println("Enter your age"); Scanner s=new Scanner(System.in); a=s.nextInt(); try { CheckAge ca=new CheckAge(); ca.verify(a); } catch(AgeException ae) { System.err.println("Age should not be -ve"); } catch(Exception e) { System.err.println(e); } } }
Compile: javac VerifyAgeException.java
finally Block in Exception Handling
Inside finallyblock we write the block of statements which will relinquish (released or close or terminate) the resource (file or database) where data store permanently.
finally block important points
- Finally block will execute compulsory
- Writing finally block is optional.
- You can write finally block for the entire java program
- In some of the circumstances one can also write try and catch block in finally block.
Example
class ExceptionDemo { public static void main(String[] args) { int a=10, ans=0; try { ans=a/0; } catch (Exception e) { System.out.println("Denominator not be zero"); } finally { System.out.println("I am from finally block"); } } }
Output
Denominator not be zero I am from finally block
Difference Between Throw and Throws Keyword
throw
throw is a keyword in java language which is used to throw any user defined exception to the same signature of method in which the exception is raised.Note: throw keyword always should exist within method body.whenever method body contain throw keyword than the call method should be followed by throws keyword.Syntax
class className { returntype method(...) throws Exception_class { throw(Exception obj) } }throws
throws is a keyword in java language which is used to throw the exception which is raised in the called method to it's calling method throws keyword always followed by method signature.Example
returnType methodName(parameter)throws Exception_class.... { ..... }
No comments:
Post a Comment